NEW React 19 Updates Are Amazing!
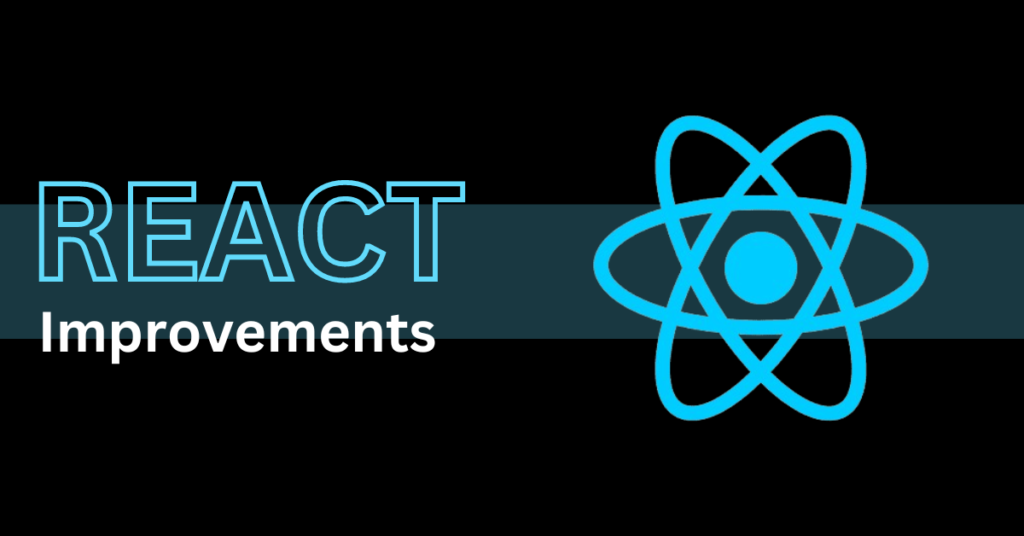
ReactJS stands out as a the most popular UI library within the realm of front-end development. In this article we gonna go through the main changes of react 19. I’ll try to keep it as simple as possible and straightforward. Let’s get started!
Table of Contents
Toggle1. React Compiler – React Forget
As we all know, one of the reasons that react is so popular, is its speed. But now React will have its own compiler called React Forget that will transform react into a high level programming language and it’s in our case JavaScript, resulting a significant speed, react was fast and it’ll become even faster.
Currently, React doesn’t re-render automatically when its state changes. To make it work better and faster, you can use some special tools like useMemo(), useCallback(), and memo. React’s team thought this was a good middle ground between doing things manually and letting React handle it all by itself. They wanted React to be smarter about when to re-render.
Then React team realized that the community has a hard time to find where to add these tools or to debug, so they decided to give react its own compiler which will be doing the job and there will be no longer a use of useMemo(), useCallback() and memo. React will decide automatically how and when to change the state and update the UI.
2. Server Components 🔥
Normally, React components run on the client side. React is introducing the concept of runing these components on the server side. Resulting in proving more content to web crawlers, faster initial loading page. That means instead of using packages to handle title and description in each page and use a lot of repetitive code, React will have its own built-in support for <title> <meta> and metadata <link> tags anywhere in your components.
As for the Directives, components are by default client side, but using ‘use server’ in the top of your application, will make your component run on the server side. By using this directive, you’ll be able to use the actions.
3. Actions
Actions are a new way to handle things like forms on your website. They make it easy to change your page when someone submits a form, without making things too complicated. This is really helpful for keeping your website simple and easy for people to use.
Actions allow you to connect actions with the HTML tag <form/>. In simpler words, you can use Actions instead of the onSubmit event. These actions are just attributes of HTML forms.
Before Actions
In the first example, we gonna be using just the usual react code that we see in the form.
<form onSubmit={submitFn}>
<input type="text" name="username" />
<button type="submit">Submit</button>
</form>
After Actions
In the following we will drop the onSubmit and use the action attribute pretty much like what we used to do in PHP if you are familiar with it. Both sync and async operations can be executed smoothly. Let’s see how it works!
"use server"
const submitFn= async (userData) => {
const newUser = {
username: userData.get('username'),
}
console.log(newUser)
}
const UserForm = () => {
return <form action={submitFn}>
<div>
<label>Username</label>
<input type="text" name='username'/>
</div>
<button type='submit'>Submit</button>
</form>
}
export default UserForm ;
In the above code, we called the directive “use server” to say that this component will run in the server side, then we created the async function submitFn and we get the user info in this case the username just by getting the value from the name attribute without using the usual onChange on every input, that means less code to deal with.
4. Asset Loading
The new React 19 feature helps images and files load faster. It begins loading them in the background while people are looking at the current page. This means there’s less waiting time when you move to a new page.
Some of you might notice, that sometimes when you load your React application, you see a flicker/flash of unstyled elements and that due to the timing it takes for css stylesheets and images to be loaded.
Well, Now! with React 19 you’ll no longer encounter this issue, which means a better user experience! Amazing right?
React will ensure that the view is displayed only after everything is loaded properly.
5. New React Hooks
The new use()
hook
This new hook will simply the use of other many operations, like fetching data for example. The following is how we would normally use to fetch some data.
import {useEffect, useState} from "react"
const UserComponent = () => {
const [users, setUsers] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchUsers= async () => {
try {
const res = await fetch('https://jsonplaceholder.typicode.com/users');
const data = await res.json();
setUsers(data);
setLoading(false);
} catch (error) {
console.error(error);
} finally {
setLoading(false);
}
};
fetchUsers();
}, []);
return (<></>)
}
Now I’m gonna show you how we simplify this using the new use() hook.
import { use } from 'react';
const fetchUsers = async () => {
const res = await fetch('https://jsonplaceholder.typicode.com/users');
return res.json();
};
const UserComponent = () => {
const users = use(fetchUsers());
return (<></>)
}
And just like this, you can use the users value just like in the first state example as for loading we can use Suspense to for te loading.
In the other hand, use() will also be used instead of useContext(). Shown in the following example:
import { createContext, useState, use } from 'react';
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
const Card = () => {
// use Hook()
const { theme, toggleTheme } = use(ThemeContext);
return (
<div
className={`p-4 rounded-md ${
theme === 'light' ? 'bg-white' : 'bg-gray-800'
}`}
>
<h1
className={`my-4 text-xl ${
theme === 'light' ? 'text-gray-800' : 'text-white'
}`}
>
Theme Card
</h1>
<p className={theme === 'light' ? 'text-gray-800' : 'text-white'}>
Hello!! use() hook
</p>
<button
onClick={toggleTheme}
className='bg-blue-500 hover:bg-blue-600 text-white rounded-md mt-4 p-4'
>
{theme === 'light' ? 'Switch to Dark Mode' : 'Switch to Light Mode'}
</button>
</div>
);
};
const Theme = () => {
return (
<ThemeProvider>
<Card />
</ThemeProvider>
);
};
export default Theme
The useFormStatus()
hook
useFormStatus
is a Hook that gives you status information of the last form submission.
const { pending, data, method, action } = useFormStatus();
This hook will help you get more information about the form you are trying to submit. For example, if it’s pending it will return true, otherwise it will be false.
data contains the data the parent form is submitting.
Conclusion
That’s a quick walk through of some of the features that are coming with the new update of React 19, there are still a lot coming on or that I didn’t mention you can check their official website in this link.